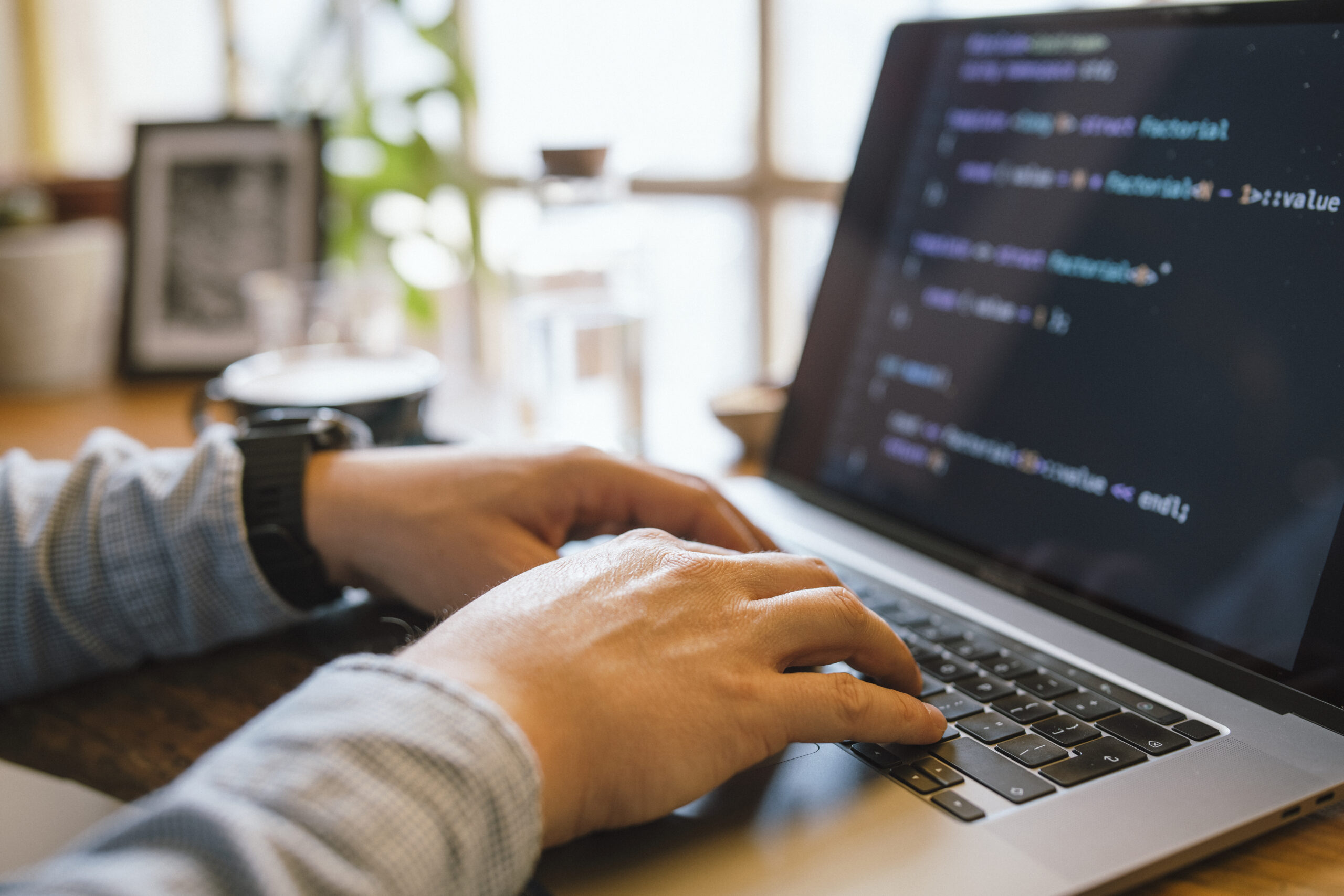
Debugging is Just about the most important — yet typically ignored — abilities in a very developer’s toolkit. It is not pretty much fixing broken code; it’s about understanding how and why matters go Completely wrong, and Mastering to Assume methodically to resolve difficulties effectively. No matter if you are a beginner or maybe a seasoned developer, sharpening your debugging competencies can save hours of frustration and significantly boost your productiveness. Listed here are numerous techniques to aid developers level up their debugging video game by me, Gustavo Woltmann.
Master Your Instruments
One of several quickest approaches developers can elevate their debugging competencies is by mastering the instruments they use daily. Though composing code is a single A part of growth, recognizing how to communicate with it properly during execution is equally important. Modern day progress environments occur Geared up with potent debugging capabilities — but numerous developers only scratch the surface of what these tools can perform.
Take, for instance, an Built-in Enhancement Atmosphere (IDE) like Visual Studio Code, IntelliJ, or Eclipse. These tools permit you to set breakpoints, inspect the worth of variables at runtime, move through code line by line, and even modify code over the fly. When made use of correctly, they Permit you to observe exactly how your code behaves during execution, and that is priceless for monitoring down elusive bugs.
Browser developer instruments, for example Chrome DevTools, are indispensable for entrance-conclude developers. They assist you to inspect the DOM, keep an eye on community requests, look at true-time overall performance metrics, and debug JavaScript from the browser. Mastering the console, sources, and network tabs can transform annoying UI issues into workable tasks.
For backend or technique-stage builders, resources like GDB (GNU Debugger), Valgrind, or LLDB supply deep control around operating procedures and memory administration. Studying these instruments can have a steeper Finding out curve but pays off when debugging performance difficulties, memory leaks, or segmentation faults.
Over and above your IDE or debugger, come to be comfortable with Variation Command techniques like Git to be aware of code historical past, locate the exact moment bugs were introduced, and isolate problematic modifications.
Ultimately, mastering your tools indicates going beyond default configurations and shortcuts — it’s about creating an intimate understanding of your improvement setting to ensure when troubles come up, you’re not lost in the dark. The higher you recognize your equipment, the more time you may invest fixing the particular issue rather than fumbling by the process.
Reproduce the condition
Among the most vital — and infrequently ignored — ways in powerful debugging is reproducing the problem. In advance of jumping into your code or producing guesses, builders want to make a consistent surroundings or circumstance where by the bug reliably seems. With out reproducibility, repairing a bug becomes a sport of probability, often leading to squandered time and fragile code improvements.
The initial step in reproducing a problem is accumulating just as much context as possible. Inquire queries like: What actions brought about The problem? Which setting was it in — improvement, staging, or manufacturing? Are there any logs, screenshots, or mistake messages? The greater element you might have, the less difficult it gets to isolate the exact conditions less than which the bug takes place.
When you finally’ve collected sufficient info, try to recreate the problem in your neighborhood natural environment. This might necessarily mean inputting a similar knowledge, simulating related user interactions, or mimicking procedure states. If The problem appears intermittently, take into consideration producing automated assessments that replicate the sting scenarios or state transitions involved. These assessments not only enable expose the condition but will also reduce regressions Later on.
Often, the issue can be atmosphere-distinct — it'd happen only on specific running systems, browsers, or below distinct configurations. Utilizing resources like virtual devices, containerization (e.g., Docker), or cross-browser tests platforms is usually instrumental in replicating this kind of bugs.
Reproducing the challenge isn’t only a action — it’s a mindset. It demands tolerance, observation, and a methodical tactic. But as you can regularly recreate the bug, you are presently halfway to repairing it. By using a reproducible circumstance, You may use your debugging applications more effectively, check potential fixes securely, and communicate a lot more Obviously along with your crew or end users. It turns an abstract grievance into a concrete challenge — Which’s where by developers thrive.
Read and Understand the Mistake Messages
Mistake messages will often be the most beneficial clues a developer has when a thing goes Improper. As opposed to viewing them as irritating interruptions, developers should really master to deal with error messages as direct communications within the process. They generally inform you what exactly occurred, where it transpired, and often even why it happened — if you know the way to interpret them.
Start off by looking at the concept very carefully and in total. Quite a few developers, particularly when beneath time tension, glance at the 1st line and straight away begin building assumptions. But further while in the mistake stack or logs may well lie the accurate root cause. Don’t just copy and paste mistake messages into search engines like yahoo — go through and fully grasp them initial.
Split the mistake down into sections. Could it be a syntax mistake, a runtime exception, or a logic mistake? Will it point to a certain file and line selection? What module or functionality activated it? These issues can manual your investigation and stage you toward the accountable code.
It’s also helpful to be familiar with the terminology from the programming language or framework you’re utilizing. Mistake messages in languages like Python, JavaScript, or Java frequently abide by predictable patterns, and learning to recognize these can substantially quicken your debugging system.
Some mistakes are obscure or generic, and in Individuals circumstances, it’s important to examine the context in which the error happened. Check connected log entries, input values, and recent variations inside the codebase.
Don’t ignore compiler or linter warnings possibly. These typically precede larger sized problems and supply hints about possible bugs.
Ultimately, error messages will not be your enemies—they’re your guides. Learning to interpret them accurately turns chaos into clarity, serving to you pinpoint issues a lot quicker, reduce debugging time, and become a much more effective and assured developer.
Use Logging Correctly
Logging is Among the most impressive tools in a developer’s debugging toolkit. When utilized efficiently, it provides actual-time insights into how an software behaves, serving to you have an understanding of what’s going on underneath the hood without having to pause execution or action from the code line by line.
A fantastic logging tactic starts off with recognizing what to log and at what stage. Prevalent logging degrees include things like DEBUG, Details, WARN, ERROR, and FATAL. Use DEBUG for detailed diagnostic information and facts all through enhancement, Facts for basic activities (like productive begin-ups), Alert for opportunity concerns that don’t break the applying, Mistake for true issues, and Lethal if the process can’t keep on.
Stay away from flooding your logs with excessive or irrelevant details. An excessive amount logging can obscure crucial messages and slow down your process. Target crucial events, point out adjustments, enter/output values, and significant choice details with your code.
Format your log messages Plainly and regularly. Include things like context, for example timestamps, ask for IDs, and function names, so it’s much easier to trace troubles in distributed programs or multi-threaded environments. Structured logging (e.g., JSON logs) will make it even simpler to parse and filter logs programmatically.
During debugging, logs Enable you to track how variables evolve, what problems are met, and what branches of logic are executed—all with no halting This system. They’re Particularly important in creation environments where by stepping by means of code isn’t probable.
Furthermore, use logging frameworks and tools (like Log4j, Winston, or Python’s logging module) that guidance log rotation, filtering, and integration with monitoring dashboards.
Eventually, intelligent logging is about balance and clarity. By using a effectively-assumed-out website logging method, you may reduce the time it will take to identify challenges, attain deeper visibility into your programs, and Enhance the In general maintainability and reliability of the code.
Imagine Similar to a Detective
Debugging is not just a specialized process—it is a method of investigation. To successfully discover and resolve bugs, builders ought to approach the method just like a detective resolving a secret. This mindset will help break down sophisticated difficulties into workable pieces and follow clues logically to uncover the root trigger.
Start by gathering evidence. Look at the signs of the challenge: mistake messages, incorrect output, or performance issues. Just like a detective surveys a crime scene, collect as much relevant information as you are able to with out jumping to conclusions. Use logs, test cases, and person experiences to piece alongside one another a transparent photo of what’s happening.
Upcoming, variety hypotheses. Inquire your self: What might be creating this behavior? Have any variations a short while ago been built to your codebase? Has this challenge transpired just before under similar instances? The target is usually to narrow down possibilities and detect probable culprits.
Then, take a look at your theories systematically. Make an effort to recreate the issue inside a managed setting. Should you suspect a specific purpose or element, isolate it and validate if The problem persists. Like a detective conducting interviews, talk to your code inquiries and Allow the effects direct you closer to the reality.
Spend shut focus to small facts. Bugs typically hide from the minimum envisioned spots—like a lacking semicolon, an off-by-one mistake, or perhaps a race affliction. Be comprehensive and client, resisting the urge to patch the issue devoid of totally being familiar with it. Short-term fixes may well hide the true problem, only for it to resurface afterwards.
Finally, hold notes on what you experimented with and learned. Just as detectives log their investigations, documenting your debugging course of action can help save time for future troubles and assistance Other individuals fully grasp your reasoning.
By considering just like a detective, builders can sharpen their analytical competencies, method troubles methodically, and become more effective at uncovering hidden difficulties in complicated techniques.
Produce Checks
Writing exams is one of the best solutions to improve your debugging abilities and Total progress performance. Checks not only assist catch bugs early but in addition serve as a safety Internet that provides you self confidence when earning variations to your codebase. A nicely-tested application is easier to debug because it permits you to pinpoint just the place and when a challenge happens.
Begin with unit exams, which give attention to personal functions or modules. These little, isolated tests can rapidly reveal whether a specific bit of logic is Doing the job as predicted. When a exam fails, you immediately know where to look, noticeably lessening enough time put in debugging. Unit tests are Primarily handy for catching regression bugs—difficulties that reappear soon after Formerly becoming fixed.
Future, combine integration tests and end-to-conclusion assessments into your workflow. These assist ensure that many portions of your application do the job jointly easily. They’re particularly handy for catching bugs that take place in complex units with a number of components or products and services interacting. If anything breaks, your tests can inform you which A part of the pipeline unsuccessful and below what disorders.
Composing tests also forces you to definitely think critically regarding your code. To test a attribute adequately, you will need to understand its inputs, predicted outputs, and edge cases. This amount of comprehending Obviously leads to higher code construction and much less bugs.
When debugging an issue, composing a failing exam that reproduces the bug could be a robust first step. After the take a look at fails regularly, it is possible to focus on repairing the bug and enjoy your test move when The problem is fixed. This method makes sure that a similar bug doesn’t return in the future.
In a nutshell, producing checks turns debugging from a aggravating guessing video game right into a structured and predictable process—aiding you capture additional bugs, faster and even more reliably.
Just take Breaks
When debugging a difficult issue, it’s simple to become immersed in the challenge—observing your display screen for several hours, trying Answer right after Remedy. But One of the more underrated debugging tools is simply stepping away. Taking breaks helps you reset your mind, decrease disappointment, and sometimes see the issue from a new perspective.
When you're as well close to the code for as well lengthy, cognitive fatigue sets in. You may begin overlooking apparent mistakes or misreading code that you simply wrote just hours before. During this point out, your Mind gets significantly less productive at issue-solving. A brief stroll, a coffee break, or even switching to another undertaking for ten–15 minutes can refresh your concentrate. Many builders report obtaining the basis of a problem when they've taken time and energy to disconnect, allowing their subconscious function in the history.
Breaks also support avoid burnout, Particularly during extended debugging periods. Sitting before a display, mentally stuck, is not simply unproductive but in addition draining. Stepping away helps you to return with renewed Strength along with a clearer mentality. You could possibly instantly observe a missing semicolon, a logic flaw, or maybe a misplaced variable that eluded you prior to.
For those who’re caught, a good guideline is to established a timer—debug actively for forty five–60 minutes, then have a 5–ten moment split. Use that point to move all over, stretch, or do anything unrelated to code. It may come to feel counterintuitive, especially beneath limited deadlines, nevertheless it basically results in a lot quicker and more effective debugging In the long term.
In short, using breaks is not really a sign of weak point—it’s a smart approach. It presents your brain Room to breathe, increases your perspective, and aids you stay away from the tunnel eyesight that often blocks your progress. Debugging can be a psychological puzzle, and rest is part of fixing it.
Study From Every Bug
Each and every bug you come upon is more than just A brief setback—It is really an opportunity to develop like a developer. Irrespective of whether it’s a syntax error, a logic flaw, or perhaps a deep architectural situation, each can train you a little something beneficial should you make time to replicate and review what went Completely wrong.
Start by asking your self several essential inquiries when the bug is solved: What brought about it? Why did it go unnoticed? Could it are actually caught before with improved tactics like device tests, code assessments, or logging? The responses normally expose blind places as part of your workflow or knowledge and assist you Establish much better coding behaviors transferring ahead.
Documenting bugs can be a fantastic routine. Preserve a developer journal or sustain a log where you note down bugs you’ve encountered, the way you solved them, and That which you uncovered. After a while, you’ll start to see patterns—recurring challenges or prevalent problems—which you can proactively stay away from.
In team environments, sharing Anything you've figured out from a bug along with your peers is often Specially effective. Whether or not it’s via a Slack concept, a short produce-up, or A fast information-sharing session, helping Many others stay away from the exact same difficulty boosts crew efficiency and cultivates a more robust Understanding society.
Far more importantly, viewing bugs as lessons shifts your way of thinking from disappointment to curiosity. Instead of dreading bugs, you’ll start off appreciating them as important portions of your advancement journey. In fact, several of the best developers are not the ones who generate excellent code, but those who continually master from their blunders.
Eventually, Every bug you deal with adds a fresh layer towards your skill set. So future time you squash a bug, take a second to replicate—you’ll occur away a smarter, far more able developer due to it.
Conclusion
Improving upon your debugging abilities normally takes time, observe, and patience — nevertheless the payoff is large. It will make you a more productive, self-confident, and able developer. The next time you're knee-deep within a mysterious bug, recall: debugging isn’t a chore — it’s a possibility to become much better at Whatever you do.